Mastering Getter and Setter Methods in Ruby: Enhance Your Object-Oriented Programming
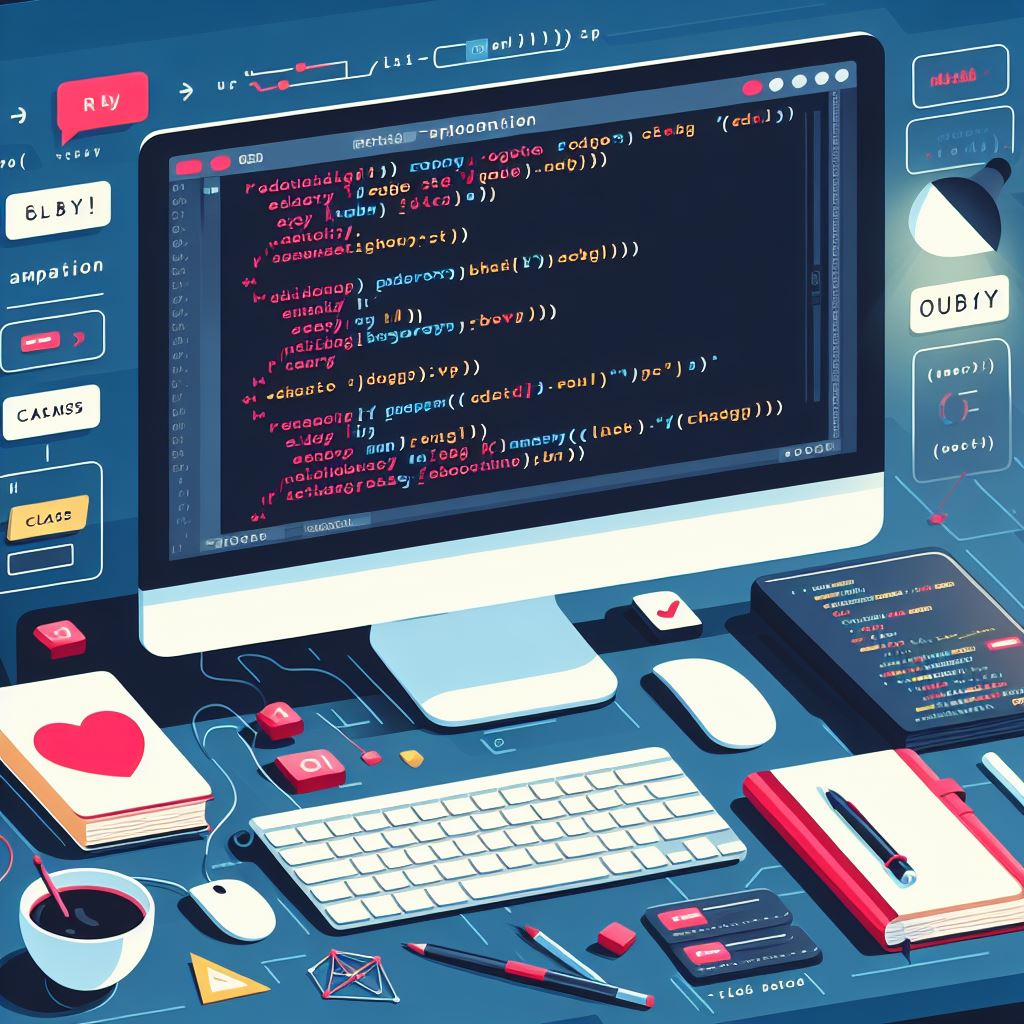
In the world of object-oriented programming, mastering getter and setter methods is important to effectively manage the state of objects. Ruby, with its most powerful weapon, elegant syntax, and other powerful features, offers a unique approach to these getter and setter methods. This article deep dives into the topic of getter and setter methods in Ruby.
What is a getter method?
If we need to access a value of a private variable from a class we use a getter method which is commonly known as accessor.
- accessor or getter
What is a settter method?
When we need to change the value of a variable, that is defined inside a class, we use a mutator method commonly known as setter method or writer method.
- mutator or setter or writer
Demonstration
To demonstrate this we will define a class named Car.
class Car
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
end
Now we make a new instance of the class named Car.
car = Car.new("Focus","Ford","White")
Accessing variables
How do we get the values of the instance variables from the car object?
One way is to use the instance_variable_get
method like this
puts "Car model is " + car.instance_variable_get(:@model)
puts "Car company is " + car.instance_variable_get(:@company)
puts "Car color is " + car.instance_variable_get(:@color)
Defining a custom getter method
A much simpler solution is to define a new getter method for each attribute.
class Car
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
def model
@model
end
def company
@company
end
def color
@color
end
end
now we make a new instance of the class named Car
car = Car.new("Focus","Ford","White")
now we get the instance variable values using getter methods
puts "Car model is " + car.model
puts "Car company is " + car.company
puts "Car color is " + car.color
Using Ruby’s built-in attr_reader
method
It is much easier to get the value of the color instance variable with car.color
than car.instance_variable_get(:@color)
The best way to make a getter method is to use the built-in Ruby attr_reader
method which automatically creates getter methods for provided attributes
class Car
attr_reader :model, :company, :color
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
end
Now we make a new instance of the class named Car
car = Car.new("Focus","Ford","White")
Now we get the instance variable values using attr_reader
puts "Car model is " + car.model
puts "Car company is " + car.company
puts "Car color is " + car.color
Setting variables
To set instance variables inside the car object we can use the instance_variable_set
method
First, define a class named Car
class Car
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
end
now we make an new instance of the class named Car
car = Car.new("Focus","Ford","White")
to change the values of the color instance variable use instance_variable_set
method
car.instance_variable_set(:@color,"Tango")
Defining a custom setter method
or we could define a setter method
class Car
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
# We define a setter method for color attributes inside the class
def color=(new_color)
@color = new_color
end
end
car = Car.new("Focus","Ford","White")
Let’s display the current values of instance variables
puts car.inspect
let’s change the color of the car using the setter method
car.color = "Tango"
let us check if the color was changed
puts car.inspect
the color of the car now is Tango instead White
Using Ruby’s built in attr_writer
method
Ruby provides the attr_writer
method which provides setter methods for provided attributes, for example
class Car
attr_writer :model, :company, :color
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
end
car = Car.new("Focus","Ford","White")
Change instance_variable
color
car.color = "Tango"
Check if the value was changed
car.inspect
Define getters and setters in the same class
One way using attr_reader
and attr_writer
methods.
class Car
attr_reader :model, :company, :color
attr_writer :model, :company, :color
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
end
The easiest way is using the attr_accessor
method.
class Car
attr_accessor :model, :company, :color
def initialize(model, company, color)
@model = model
@company = company
@color = color
end
end